- Patryk Gańczarek-Rał
- Read in 4 min.
If you’re still running your .NET Core app on an older version of the framework, it may be time to upgrade to .NET Core 7. In this article, I’ll explain why it’s worth making the switch. You will learn about some of the performance enhancements that .NET Core 7 offers.
.NET Core Support Policies
First, let’s take a quick look at .NET Core’s support policies. You can choose between Long Term Support (LTS) release or Standard Term Support (STS) release. The only difference between them is the length of support. LTS gets free support and patches for 3 years and STS for 18 months. .NET 5 has reached the end for that last year, and the current LTS release of .NET Core, which is version 6, will be supported just until November 2024. If you plan to use .NET in your new project or are already using an older version, you may want to consider upgrading to .NET Core 7.
Why Upgrade to .NET Core 7?
The key reason to migrate your app to .NET Core 7 is performance. Over 1000 pull requests were merged into .NET Core 7, which now helps ensure code quality and reduce the risk of introducing bugs or conflicts. The number of optimizations and improvements is so enormous that we cannot describe everything. If you want to look into more technical details, see the full performance improvements description created by Microsoft. One thing is for sure – .NET 7 is crazy fast! Let’s see some examples.
LINQ
LINQ is a feature used by every .NET developer. In previous versions of .NET Core, LINQ queries could be slow and memory-intensive, especially for complex queries. However, in .NET Core 7, the LINQ engine has been optimized for performance, resulting in much faster query times and lower memory usage. Let’s look at the example given by Microsoft in their official .NET 7 performance description. Here you can see a part of it:
private static float[] CreateRandom() { var r = new Random(42); var results = new float[10_000]; for (int i = 0; i < results.Length; i++) { results[i] = (float)r.NextDouble(); } return results; } private IEnumerable<float> _floats = CreateRandom(); [Benchmark] public float Sum() => _floats.Sum(); [Benchmark] public float Average() => _floats.Average(); [Benchmark] public float Min() => _floats.Min(); [Benchmark] public float Max() => _floats.Max();
Results:
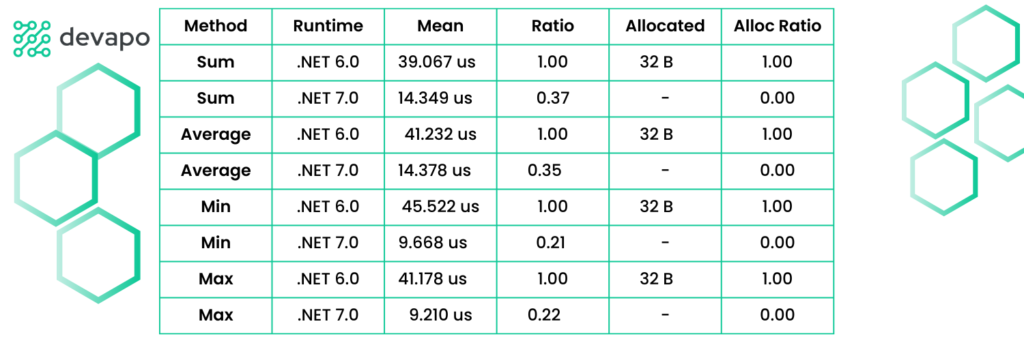
Looking at the table above, we can see how drastically the performance improved.
Improved Startup Performance
With performance being the biggest focus of .NET 7, it’s no surprise that we can see massive improvements in startup time. According to Microsoft, it can be up to 10-15% better. It means that not only the .NET 7 apps are faster, but so is their development. This can make a huge difference, especially in big and complex systems. Your businesses may stay ahead of the competition, as now you could implement new and innovative solutions to the customers way faster.
Improved performance of System.Reflection
The reflection mechanism in .NET allows for manipulating the loaded types instances and creating types dynamically. With the newest update, we can see up to 3-4 times faster results according to benchmarks provided by Microsoft.
Other benefits you will get from migrating to .NET 7
Easier Maintenance: Each new release of .NET includes security enhancements and bug fixes, which can make it easier to maintain and update your application. This can help reduce downtime and increase uptime, which can have a positive impact on your business operations.
Long-term Support: Staying up to date with the latest version of .NET ensures that your application will continue to receive official support from Microsoft. This can help ensure that your application remains stable and secure over the long term. It is especially important for businesses that rely on their applications for critical operations.
Summary
If you’re still running your .NET Core app on an older version of the framework, now is the time to upgrade to .NET Core 7. The end of support for some older versions makes it essential to upgrade to .NET Core 7 to keep your application safe and secure.
With its improved performance, new features, and enhanced security, it’s a smart choice for any application. It may not be a straightforward process, especially with the older version than 6, but it’s worth the effort. Here, at Devapo, we have a team of .NET developers who could help you with that. If you are in need, let’s talk!